5 Mistakes in PHP Programming (and How to Avoid Them)
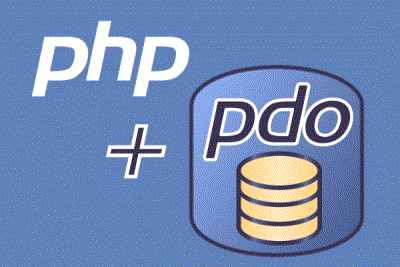
As a novice PHP programmer, there are a few mistakes that everyone makes. These are the errors that are very basic and can easily be avoided. For example, using an ‘assignment’ operator (=) when you actually intend to compare things (using the == operator). If you started out with C or C++ programming, you are most vulnerable to this mistake. Similarly, PHP is not forgiving if you ever divide a number by ‘zero’ and throws up an error immediately.
Many PHP students end up creating an infinite loop because they forget to increment the counter every time the loop repeats itself. Typo errors such as misspelling the name of a variable and forgetting to open or close a string or parentheses (brackets) properly are other common mistakes that people make in PHP coding.
Here are the five mistakes that even experienced PHP programmers make that can easily be avoided:
Not rewriting URLs
Modern-day practices require you to rewrite URLs that are clean and legible. There are various framework guides (such as Symfony and Zend) that can help you learn the art and science of writing such URLs.
A URL that uses too many variables is not easy to read…especially over the phone.
For example, a URL like
http://www.pets.com/show_a_product.php?product_id=34
can be rewritten as
http://www.pets.com/products/34/
which is cleaner, shorter, and easier to remember.
Now, if we write this URL as:
http://www.pets.com/products/rottweiler-dogs/
It will immediately give you an idea of what you will find on this page. It is an easy URL to remember and share with friends. Please note that the search engines treat hyphens as spaces and hence, they should be preferred over underscores while rewriting the URL.
Apache comes with its own URL rewriting module and is mod_rewrite enabled. For Microsoft’s server software – IIS – there are add-ons like ISAPI_Rewrite that can be used for URL rewriting.
Still Stuck with MySQL Extension
MySQL extension is now outdated. PHP 5.5.0 deprecates it and the deprecation notices appear on the top of the app. Hackers can easily access these notices using Google and find out that your site is insecure and vulnerable to attacks. Moreover, if you continue to use MySQL extension, you have a site that does not support SSL and is considered unreliable.
It is advisable to use MySQLi extension instead (or migrate onto it) as it is faster, has enhanced security and debugging features, and supports:
- prepared statements,
- procedural as well as object-oriented interface,
- stored-procedure, and
- transactions through API.
Not Using or Under-using PDO
PHP Data Objects (PDO) is a time-saving feature that allows you to fetch data directly into objects and use named parameters easily. You can use the object-oriented syntax to align your code with the popular databases like MS SQL and PostgreSQL.
Despite hundreds of tutorials available online, half of the features of PDO still remain obscure and PHP developers keep trying to reinvent something which already exists in the PDO.
PDO is a Database Access Abstraction Layer that serves as a unified interface to access many different databases and helps in automating several basic operations that need to be repeated hundreds of times when you are writing an application code. PHP assignment writing experts use PDO on a regular basis to come up with clean and secure PHP code quickly.
Skip Database Caching
Cache enhances the performance as well as the user experience of an app or a website. Caching in PHP means storing away information (such as HTML pages, web objects, and files) in the users’ local hard drive for future use.
There are three things that can be cached in PHP – content, memory, and database. To cache content, we save the final output of a specific PHP script into the file system. Since the database access is bypassed, it will load faster than the previous request.
Memory Cache is faster than caching in the file system and uses Opcode Cache, APC (which has to be installed separately), and Memcached features. While most PHP developers do content and memory caching in the application layer, they often forget to do database caching which needs to be done on the database server.
When you cache the results of the query for a database, the query will not need to be parsed again and again – and gets processed much faster.
Improper Handling of Errors
Errors pop up to signify that something somewhere is not right. When we suppress errors, we allow the app to keep running despite the potential bugs – and not do anything about it. On the other hand, too many errors on the website or the application can be too confusing and irritating for the end user.
Normally, one would recommend the developer to redirect the errors into an error log using the Php.ini file but that can slow down the website considerably – especially when there is high traffic on the site. Customized error handlers such as Papertrail (available as a PHP add-on) can be an answer to this problem. They can send the errors to the back-end and do not allow to be displayed on the screen, and end the application only if a grave error occurs. Programmers can then search for such errors later and fix them.
Remember that SQL queries are not as trustworthy as you think and can be tampered with. They can bypass standard authorization and authentication checks and even allows access to host commands at operating system levels. To avoid this, use prepared statements with bound variables whole coding (such as those provided by PDO and MySQLi). You may also use stored procedures and pre-defined cursors to abstract data access for users.